DA SQL sample (Android) (Eclipse)
The DA SQL Sample demonstrates how to use a DA SQL statement to filter the schema data. DA SQL provides a means to execute an SQL request in a safe manner against the schema data, rather than directly against the database.
Getting Started
To get started with this sample you need to have Eclipse, the Eclipse ADT plugin and the Android SDK installed. Regarding the SDK you need a minimum of one of the Android API builds, the build tools and a system image if you wish to test the sample out in the simulator.
When you load the project in Eclipse you will need to copy the com.remobjects.dataabstract.jar
and com.remobjects.sdk.jar
from where you installed Data Abstract for Java (typically "c:\Program Files (x86)\RemObjects Software\Data Abstract for Java\bin") into the "libs" folder of the project and if needed go into the project properties and add them on the "Java Build Path" tab.
Finally by default the sample interacts with the RemObjects sample server at "http://remobjects.com:8099/bin".
Running the Sample
When the sample starts it displays a ListView
which contains an expandable list of different DA SQL statements that could be executed. There is also a slider button which opens a settings view where you can change the server that the sample connects to.
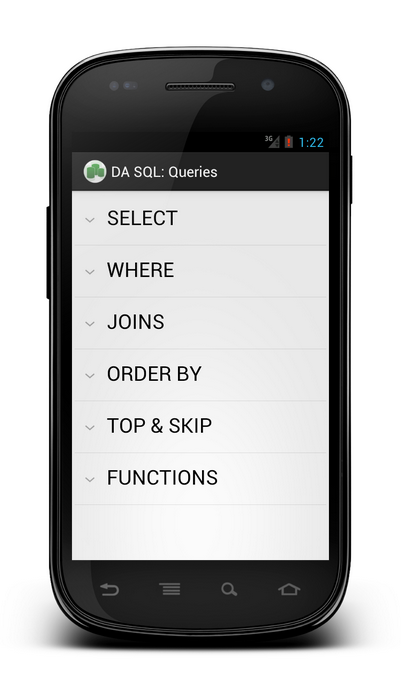
The list is split into 6 categories which emphasize a particular feature, under which is 1 or more SQL statements that can be executed. A short press on an child element will execute the request, and a long press will display the SQL that would be executed. When the request is executed the results will be displayed in a list view. To execute another request simply navigate back and choose another from the list.
This sample supports Android based tablet devices and will present the expandable list view of SQL statements in the left hand pane and the results in the right hand pane.
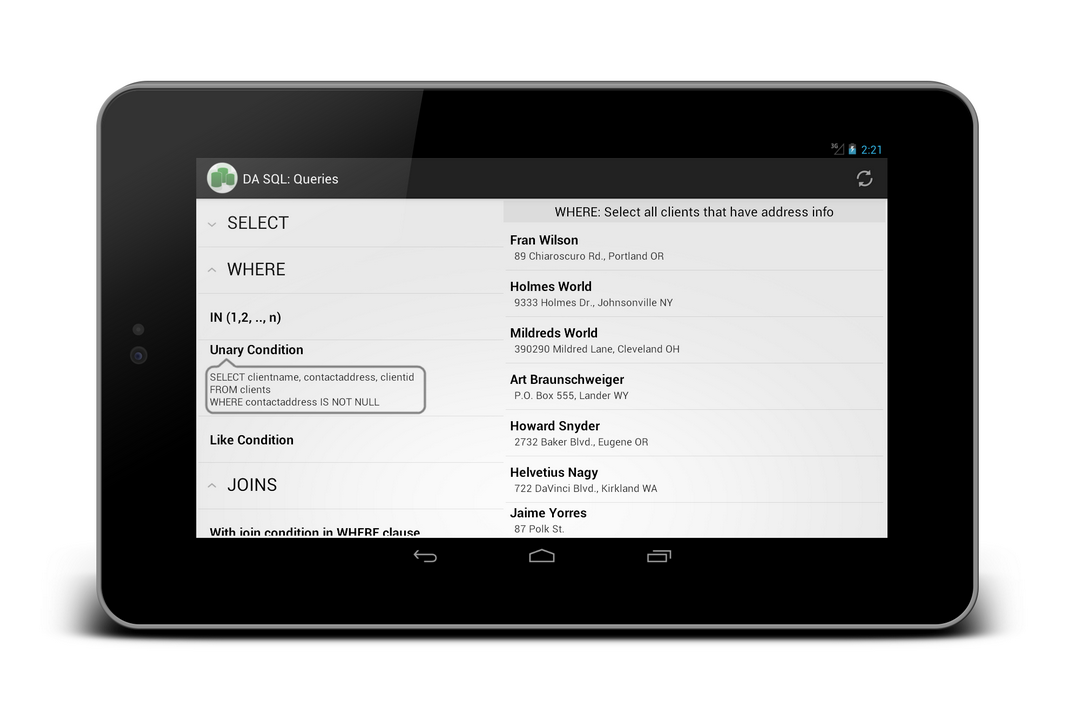
Examining the Code
The Java based sample is comprised of 6 classes that handle the UI and those functions needed to interact with the Relativity Server.
- DataModule handles initializing the basic connection to the server and creating the RemoteDataAdapter. It also registers itself to listen for changes to the preferences so that when the settings data is changed the RemoteDataAdapter is updated to reflect the new data. It also contains the DataTable to store the table data.
- TableListAdapter is a class used by ResultsFragment to display the retrieved data in the
ListView
. - SettingsActivity handles interacting with the "Preferences" subsystem for storing and retrieving data that will be used for logging in like the server url, username and password.
- MainActivity handles the main UI, reading the SQL data from the "sql.xml" resource file and presenting the retrieved results.
- ResultsActivity handles the view's UI, displaying the retrieved data via the
ResultsFragment
and displaying a title that indicates the SQL statement executed. - ResultsFragment retrieves the data from the server and creates the
TableListAdapter
used for displaying the data.
Executing a DA SQL statement
Using a DA SQL statement to retrieve data is very simple, all that is required is to pass the DA SQL statement as a string to the fillWithSqlAsync method of RemoteDataAdapter. The method takes 4 arguments, the first is the DataTable to be filled, the second is the DA SQL statement, the third is an optional array of parameters that might be needed for the DA SQL statement and the final argument is a callback instance to be executed when the fill request finishes.
//ResultsFragment.java
private void loadTable() {
ensureTable();
fTableAdapter.notifyDataSetChanged();
final Activity that = this.getActivity();
fDataModule.DataAdapter.fillWithSqlAsync(fDataModule.dataTable, fSql, null, new FillRequestTask.Callback() {
@Override
public void completed(final FillRequestTask aTask, Object aState) {
that.runOnUiThread(new Runnable() {
@Override
public void run() {
if (aTask.isFailed()) {
Throwable th = aTask.getFailureCause();
Toast.makeText(that, th.getMessage(), Toast.LENGTH_SHORT).show();
Log.e("ResultsFragment", th.getClass().getName(), th);
} else if (aTask.isCancelled()) {
Toast.makeText(that, "fill request cancelled", Toast.LENGTH_SHORT).show();
} else
fTableAdapter.notifyDataSetChanged();
}
});
}
}).execute();
}